2 Preface
“Testing leads to failure, and failure leads to understanding.”
– Burt Rutan
One of the most important skills in the 21st century is the ability to use a computer. Computers are so ubiquitous that doing anything without them — such as running a business or communicating with friends — would be inconceivable. However, we are fast moving into a time when it will no longer be sufficient to simply know how to use a computer. Instead, many roles will require that we know how to instruct the computer to get something done.
As we will soon see, a core element of computer science is about solving problems. While many other disciplines are also about attempts to solve real-world problems (for example, work on curing various diseases), there is one powerful concept that allows computer scientists to have an oversized impact on the world: that of scale.
This is because code — the instructions we write to tell the computer what to do to solve a problem — is free! We can share it with anyone and everyone, and as long as they have a computer, laptop, cellphone or any kind of computing device, they too can benefit from what we have created. Since the code is a solution to a problem, we have just allowed someone else to solve that same problem with no further work!
This is very different to the physical world. Imagine we have invented a device that can quickly purify water so that it’s safe to drink. This is undoubtedly an important problem and such a device would be immensely useful (not to mention lifesaving). However, to distribute it to people means having to manufacture it in great quantities. This requires factories, manpower, capital and so on. We need logistics to ship and distribute it. We need to worry about administrative issues and cross-border controls and a million other things, plus we need money to ensure that the enterprise doesn’t bankrupt us. None of these is a trivial matter.
By contrast, code is simply software that we can share with anyone we want by putting it on the internet. Once we’ve created the code, that’s it! We can share it (or sell it) to the world with very little effort. To create a billion copies of our program costs us nothing because it’s all virtual! When we talk of scale, we mean that if we solve a problem with code, then we can immediately reach the entire world with our solution almost single-handedly. The digital revolution of the last 50 years is, in no small part, down to the ease with which programs can proliferate. Needless to say, there is never a better time to become a skilled programmer!
2.1 Getting Started
2.1.1 HTML Canvas
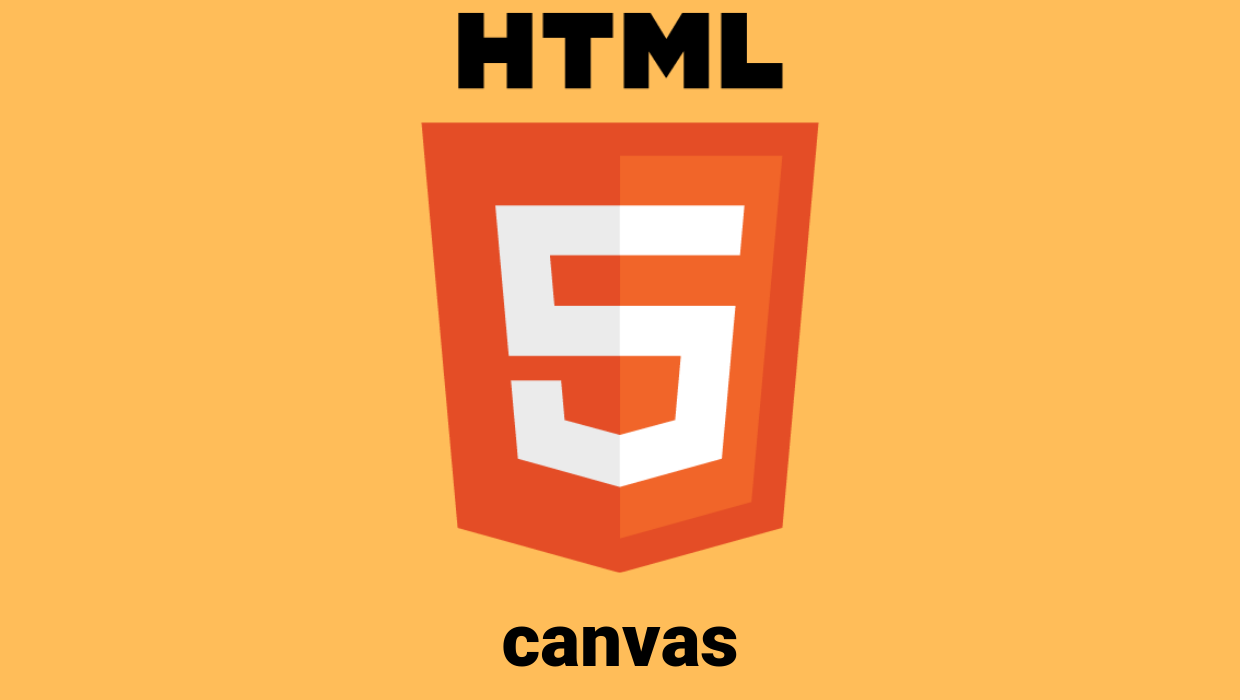
You can run an .html file by simply double clicking the file. If not you can also right-click ‘open with’ and select a web browser.
2.1.1.1 Debugging
To Debug right click the html canvas and press inspect.
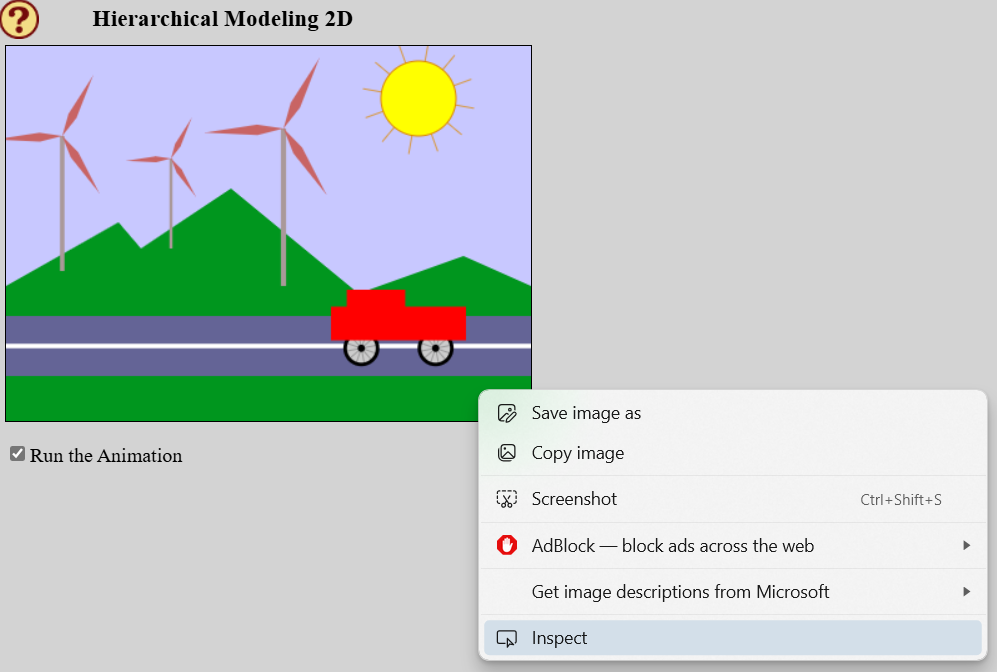
This will open up the inspection window which has a lot of information.
Most importantly though is the </> Elements
tab which contains your compiled and built html code which can be dynamically edited.
You will also find your particular script as seen below.
Lastly is the Console
tab which will contain a list of all errors similar to output you would see if there were compilation errors in C.
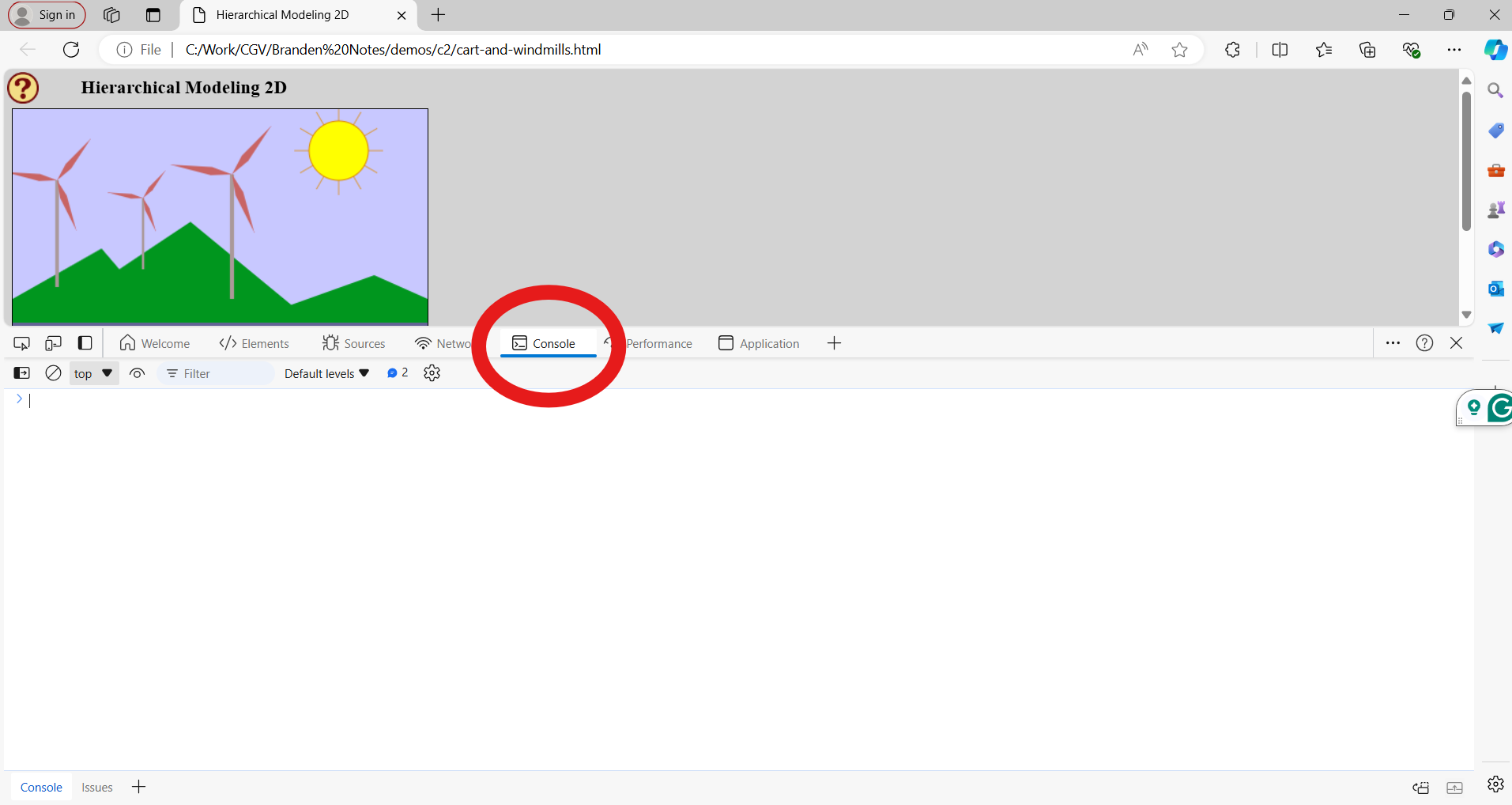
2.1.2 OpenGL
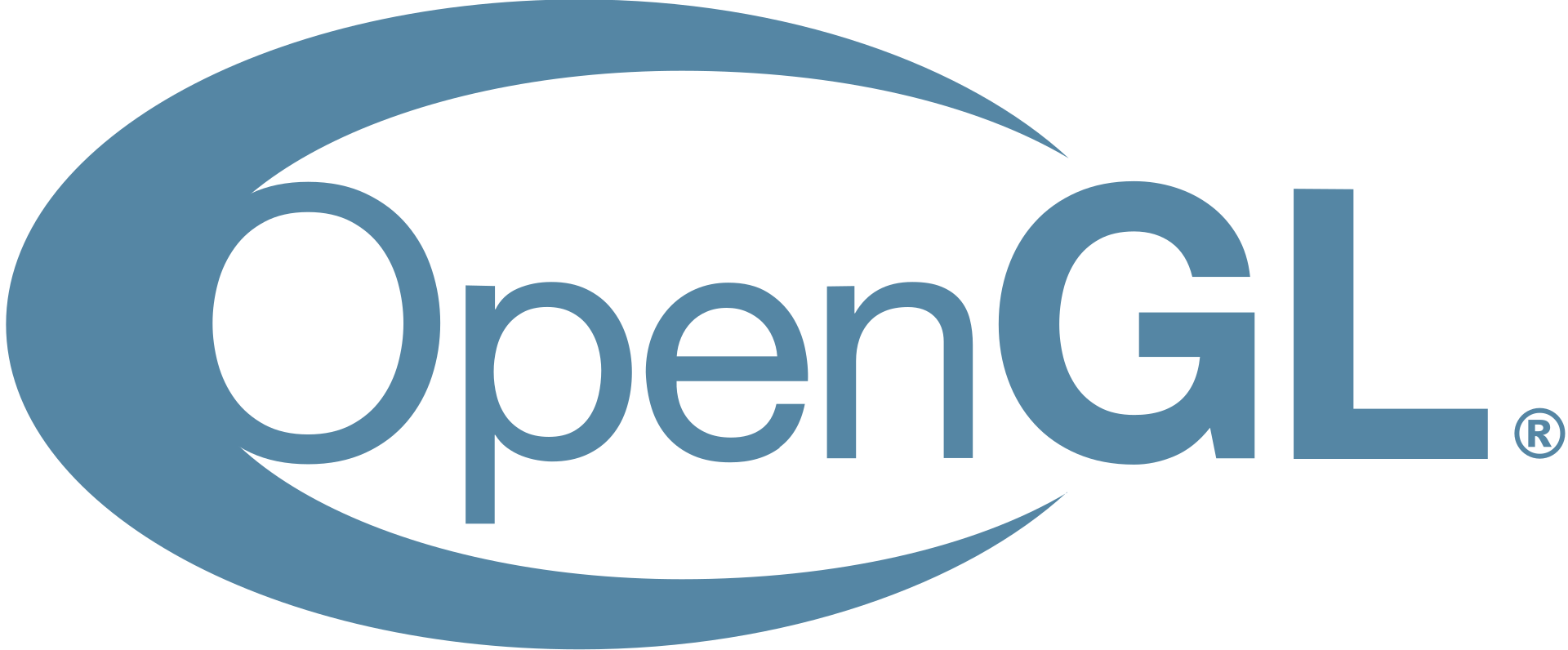
2.1.2.1 Requirements
To compile and run OpenGL code using C, you need the following:
- C Compiler: GCC, MinGW, or Xcode.
- OpenGL Libraries: Core OpenGL library and GLU (optional).
- Window Management Library: GLFW or GLUT.
- Development Environment: An IDE or a text editor with command-line tools.
2.1.2.2 Installation
-
Install a Compiler
- Linux: GCC is usually pre-installed. If not, install it using a package manager (e.g., sudo apt-get install build-essential).
- Windows: Install MinGW or MSYS2 which includes GCC.
- macOS: Install Xcode from the App Store.
-
Install OpenGL and Auxiliary Libraries
-
Linux: Install using a package manager
sudo apt-get install libgl1-mesa-dev libglu1-mesa-dev freeglut3-dev
-
Windows: OpenGL comes with the graphics driver.
- Download GLUT or GLFW from their respective websites.
-
macOS: OpenGL is pre-installed.
- Install GLFW using Homebrew (brew install glfw).
-
Linux: Install using a package manager
-
Set Up Your Development Environment
- Use VsCode
- Write and Compile Your Code
Linux/MacOS
MacOS
Please note you will need to use #include <GLUT/glut.h>
for MacOS instead of #include <GL/glut.h>
in Linux.
However, this is more important in the following section that contains the example.
gcc -o opengl_example opengl_example.c -framework GLUT -framework OpenGL -framework Cocoa
./opengl_example
Windows
The following is an example program with the correct corresponding output, do not worry yet if it looks confusing.
#include <GL/glut.h>
GLfloat vertices[] = {
// Triangle
-0.6f, -0.6f, 0.0f,
0.6f, -0.6f, 0.0f,
0.0f, 0.6f, 0.0f,
// Square
0.7f, -0.6f, 0.0f,
1.7f, -0.6f, 0.0f,
1.7f, 0.6f, 0.0f,
0.7f, 0.6f, 0.0f
};
GLubyte indices[] = {
0, 1, 2, // Triangle
3, 4, 5, 6 // Square
};
GLfloat colors[] = {
1.0f, 0.0f, 0.0f, // Red
0.0f, 1.0f, 0.0f, // Green
0.0f, 0.0f, 1.0f, // Blue
1.0f, 1.0f, 0.0f, // Yellow
1.0f, 1.0f, 0.0f, // Yellow
1.0f, 1.0f, 0.0f, // Yellow
1.0f, 1.0f, 0.0f // Yellow
};
void display() {
glClear(GL_COLOR_BUFFER_BIT);
// Using glDrawArrays for the triangle
glEnableClientState(GL_VERTEX_ARRAY);
glEnableClientState(GL_COLOR_ARRAY);
glVertexPointer(3, GL_FLOAT, 0, vertices);
glColorPointer(3, GL_FLOAT, 0, colors);
glDrawArrays(GL_TRIANGLES, 0, 3);
glDisableClientState(GL_VERTEX_ARRAY);
glDisableClientState(GL_COLOR_ARRAY);
//glClear(GL_COLOR_BUFFER_BIT);
// Using glDrawElements for the square
glEnableClientState(GL_VERTEX_ARRAY);
glEnableClientState(GL_COLOR_ARRAY);
glVertexPointer(3, GL_FLOAT, 0, vertices);
glColorPointer(3, GL_FLOAT, 0, colors);
glDrawElements(GL_QUADS, 4, GL_UNSIGNED_BYTE, indices + 3);
glDisableClientState(GL_VERTEX_ARRAY);
glDisableClientState(GL_COLOR_ARRAY);
glutSwapBuffers();
}
void reshape(int w, int h) {
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(-1, 2, -1, 1); // Adjusted to fit both shapes
glMatrixMode(GL_MODELVIEW);
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB);
glutInitWindowSize(800, 600);
glutCreateWindow("glDrawArrays and glDrawElements Demo");
glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
glutDisplayFunc(display);
glutReshapeFunc(reshape);
glutMainLoop();
return 0;
}
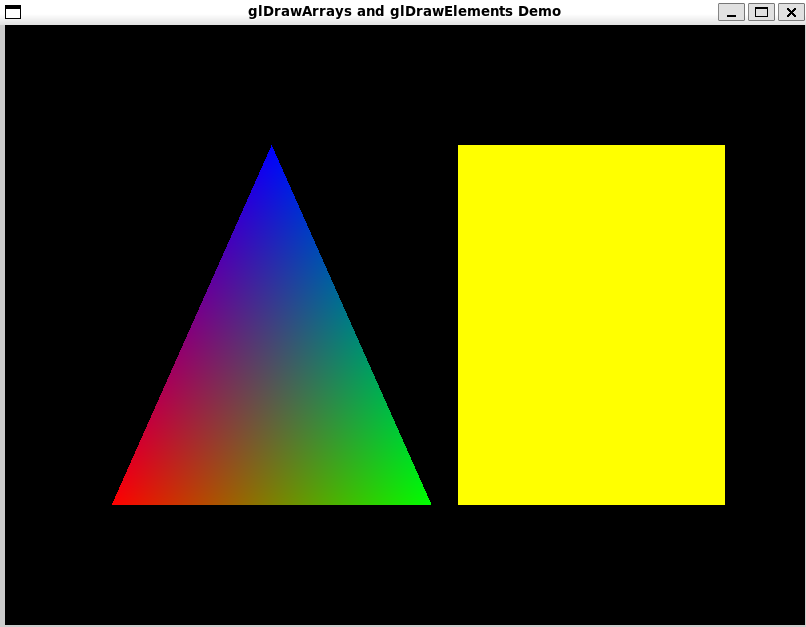
How to Use This Book
This book should be read linearly, one chapter at a time. Each chapter has associated videos that expand on some of the concepts, as well as short questions to test your understanding. Most importantly, each chapter contains links to practical exercises. As mentioned, it is impossible to learn to program by simply reading and watching videos, so these lab exercises are the most critical of all. We recommend you complete these before moving on to the next chapter, since each new chapter builds on knowledge of the previous ones.
Text Boxes
Throughout the book, a set of text boxes is used.
General Note
General notes contain relevant background information, insights, anecdotes, considerations or take-home messages pertaining to the covered topic.
Important Information
These boxes contain information on caveats, problems, drawbacks or pitfalls you have to keep in mind.
Quick Questions
After each chapter, this box will contain a few questions through which you can test your knowledge. Answers to these questions can be found at the end of the book in Appendix A.
Worked Practical
These boxes contain programming questions that you can attempt on your own. They will often be accompanied by a video demonstrating how the problem can be solved. We recommend that you first attempt the problem before watching the video. Remember, there is always more than one way to arrive at the correct answer.
Side Quest
These are random questions, tasks and digressions that you may wish to look into if you are curious. They are not necessary, but are just fun activities that you may be interested in tackling. Learning shouldn’t be about doing something because you have to.
Lab Practicals
These boxes contain links to the practical problems you’re required to solve and submit as part of the course.
Code Snippets
Throughout this book, you will see various snippets of code. You can distinguish them from regular text because they are formatted using a different font, as you can see below.
In line with the comment on interactivity being key to programming, we recommend that when you see a code snippet, don’t just read it! Instead, copy it (there is even a little icon on the right that you can click to copy it to your clipboard) and try it out for yourself — play around and see if you can slightly modify it to do something else!