14.28 DetermineShootAtGoalPosition
This function is used to optimize the target we kick for when shooting for a goal. The function starts by looking at a position just a bit lower down than the top post of the goal VecPosition initTarget = VecPosition(HALF_FIELD_X,HALF_GOAL_Y-0.1,0);
. We then iterate from this point checking if this target is obstructed using isThereObstructionBetweenTargetAndBall
however in this case we use 0.25
as our threshold as we want to be a lot more strict on our bounding rectangle. These rectangles can be seen in the Figures below. If that initial target is obstructed we decrease the y-coordinate by the offset
value. This process is repeated until an unobstructed target is found. When this target is found it is returned as our adjusted VecPosition
. If no target can be found we then look for a valid pass recipient using DetermineValidPassRecipient
with the pass back parameter enabled.
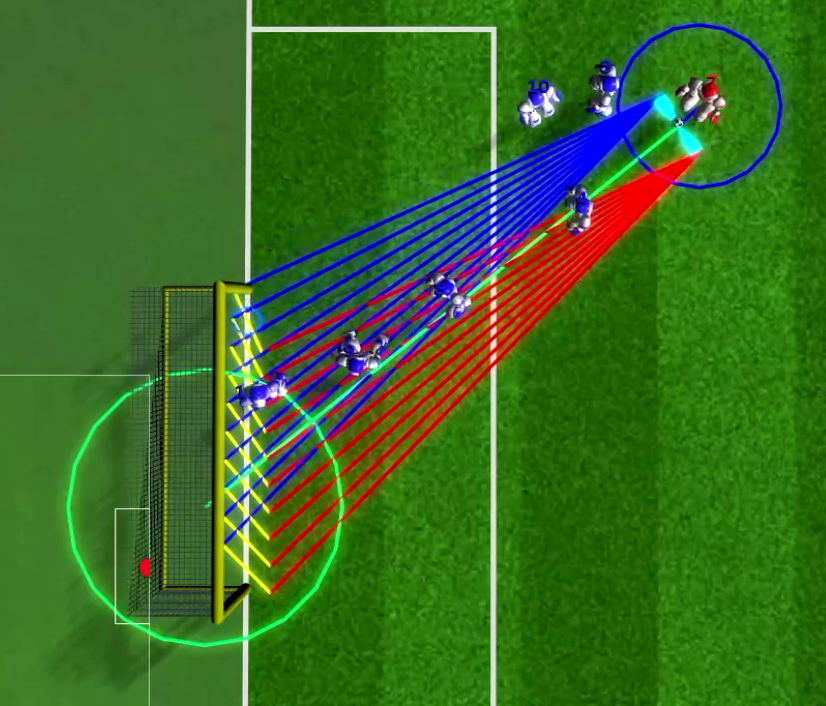
Figure 14.4: Visualisation of optimised target selection when shooting at goal depicted by small blue circle
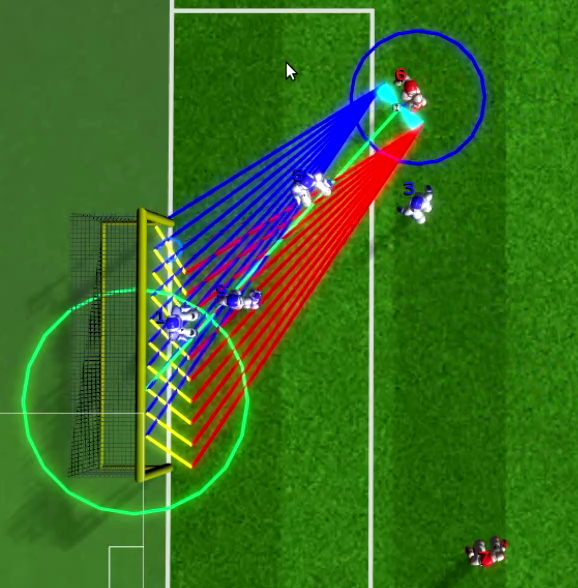
Figure 14.5: Visualisation of optimised target selection when shooting at goal depicted by small blue circle
VecPosition NaoBehavior::DetermineShootAtGoalPosition(VecPosition _myPos, int _playerNumber, vector<pair<double,int > > TeamDistToBall){
double offset = 0.2;
VecPosition initTarget = VecPosition(HALF_FIELD_X,HALF_GOAL_Y-0.1,0);
while(isThereObstructionBetweenTargetAndBall(initTarget,1,0.25)){
initTarget.setY(initTarget.getY()-offset);
if(initTarget.getY() < -HALF_GOAL_Y){
double yValue = MapValueInRangeTo(ball.getY(), -HALF_FIELD_Y, HALF_FIELD_Y, -HALF_GOAL_Y, HALF_GOAL_Y);
initTarget.setY(yValue);
return initTarget;
}
}
VecPosition Adjustedtarget = DetermineValidPassRecipient(initTarget,_myPos,_playerNumber,TeamDistToBall,true);
return Adjustedtarget;
}