14.27 DetermineValidPassRecipient
This function is utilised when the current target for a kick is obstructed, in other words if isThereObstructionBetweenTargetAndBall
returned true
, in order to determine an alternate target to kick towards. The general idea is if our original target is obstructed we check if the 4 closest teammates to the ball pass some criteria. If one of those teammates do pass all the checks we return a VecPosition
representing the position of that player so that we can pass to them. If no teammates pass the criteria we simply target slightly above or below our original VecPosition currTarget
. The criteria that needs to be passed is that if passbackallowed
is false
the teammate needs to be further up field than the player kicking. This is to ensure no back passes as it would take to long to move to the opposite side of the ball. Another criteria is that the agent needs to be further than 2 units away just so that we dont perform any very short range passes. Lastly the teammate themself needs to unobstructed and this is determined by simply reusing the isThereObstructionBetweenTargetAndBall
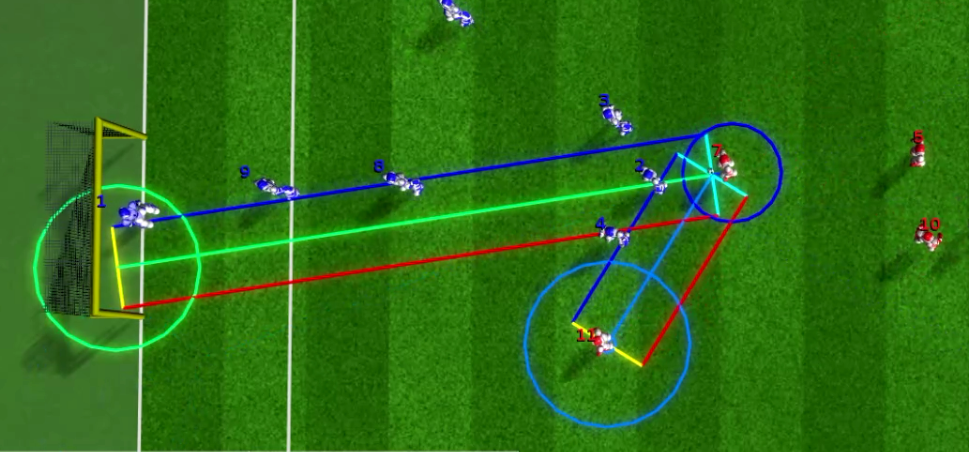
Figure 14.2: Visualisation of updated target depicted by blue circle
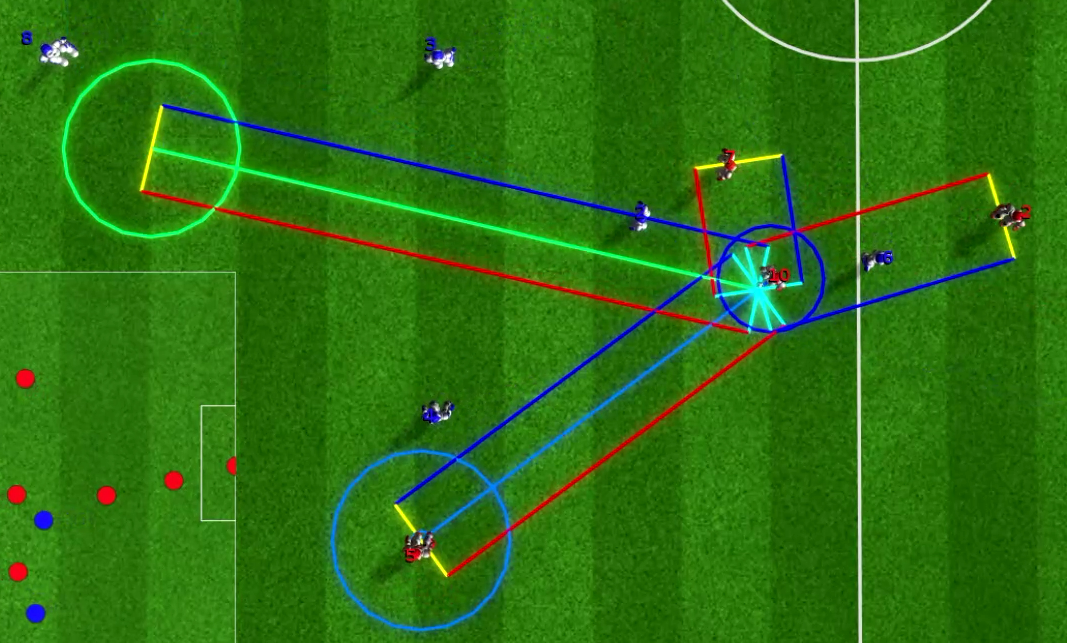
Figure 14.3: Visualisation of updated target and teammate checking
VecPosition NaoBehavior::DetermineValidPassRecipient(VecPosition currTarget, VecPosition mypos, int _playerNumber, vector<pair<double,int > > TeamDistToBall, bool passbackallowed){
if(isThereObstructionBetweenTargetAndBall(currTarget)){
bool found = false;
int currIndex = 0;
while(!found){
int pNum = TeamDistToBall[currIndex].second;
if(_playerNumber != pNum){
VecPosition tempTarget = worldModel->getWorldObject(pNum)->pos;
if(isThereObstructionBetweenTargetAndBall(tempTarget)){
}
else{
if(passbackallowed){
double distToTarget = mypos.getDistanceTo(tempTarget);
if(distToTarget>2){ // prevent very close range pass
return tempTarget;
}
}
else if(tempTarget.getX() > mypos.getX()){ //prevent back pass
double distToTarget = mypos.getDistanceTo(tempTarget);
if(distToTarget>2){ // prevent very close range pass
return tempTarget;
}
}
}
}
currIndex++;
if(currIndex>4){ // if we cant find a viable pass from the 4 closest players then move on
found = true;
}
}
if(ball.getY()>0){
currTarget.setY(currTarget.getY()-1.5);
return currTarget;
}
else{
currTarget.setY(currTarget.getY()+1.5);
return currTarget;
}
}
else{
return currTarget;
}
}